Java (programming language)
Java is an object-oriented programming language and a registered trademark of Sun Microsystems, which was acquired by Oracle in 2010. The programming language is a component of Java technology - this basically consists of the Java development tool (JDK) for creating Java programs and the Java runtime environment (JRE) for executing them. The runtime environment itself includes the virtual machine (JVM) and the libraries that come with it. Java as a programming language should not be equated with Java technology; Java runtime environments execute bytecode that can be compiled from both the Java programming language and other programming languages such as Groovy, Kotlin, and Scala. In principle, any programming language could be used as a basis for Java bytecode, but mostly no corresponding bytecode compilers exist.
Within Java technology, the Java programming language is primarily used to formulate programs. These are initially available as pure, human-understandable text, the so-called source code. This source code is not directly executable; only the Java compiler, which is part of the development tool, translates it into the machine-comprehensible Java bytecode. However, the machine that executes this bytecode is typically virtual - that is, the code is usually not executed directly by hardware (such as a microprocessor), but by corresponding software on the target platform.
The purpose of this virtualization is platform independence: The program should be able to run on any computer architecture without further modification if a suitable runtime environment is installed there. Oracle itself offers runtime environments for the operating systems Linux, macOS, Solaris and Windows. Other manufacturers have their own Java runtime environments certified for their platform. Java is also used in cars, hi-fi systems and other electronic devices.
To increase the execution speed, concepts such as just-in-time compilation and hotspot optimization are used. In terms of the actual execution process, the JVM can thus interpret the bytecode, but also compile and optimize it if necessary.
Java is one of the most popular programming languages. In the TIOBE index published since 2001, Java has always been ranked #1 or #2, competing with C. According to the RedMonk Programming Language Index 2019, Java is ranked second after JavaScript, along with Python.
Basic Concepts
The design of the Java programming language aimed mainly at five goals:
- It should be a simple, object-oriented, distributed and familiar programming language.
- It should be robust and safe.
- It should be architecture neutral and portable.
- It's supposed to be very powerful.
- It should be interpretable, parallelizable and dynamic.
Simplicity
Java is simple compared to other object-oriented programming languages such as C++ or C# because it has a reduced language scope and does not support operator overloading and multiple inheritance, for example.
Object Orientation
Java belongs to the object-oriented programming languages.
Distributed
A number of simple options for network communication, from TCP/IP protocols to remote method invocation to Web services, are provided primarily through Java's class library; the Java language itself does not include direct support for distributed execution.
Familiarity
Because of the syntactic closeness to C++, the original similarity of the class library to Smalltalk class libraries, and the use of design patterns in the class library, Java shows no unexpected effects for the experienced programmer.
Robustness
Many of the design decisions made in the definition of Java reduce the likelihood of unintended system errors; notable among these are strong typing, garbage collection, exception handling, and abandonment of pointer arithmetic.
Security
This is supported by concepts such as the class loader, which controls the secure delivery of class information to the Java Virtual Machine, and security managers, which ensure that access is only allowed to program objects for which appropriate rights are available.
Architecture neutrality
Java is designed so that the same version of a program will, in principle, run on any computer hardware, regardless of its processor or other hardware components.
Portability
In addition to being architecture neutral, Java is portable. This means that primitive data types are standardized in their size and internal representation as well as in their arithmetic behavior. For example, a float
is always an IEEE 754 float of 32 bits in length. The same applies, for example, to the class library, which can be used to create a GUI independent of the operating system.
Performance
Java has the potential to achieve better performance than languages limited to compile-time optimizations (C++, etc.) due to the run-time optimization possibility. This is countered by the overhead caused by the Java runtime environment, so that the performance of, for example, C++ programs is surpassed in some contexts, but not achieved in others.
Interpretability
Java is compiled into machine-independent bytecode, which in turn can be interpreted on the target platform. Oracle's Java Virtual Machine interprets Java bytecode before compiling and optimizing it for performance reasons.
Parallelizability
Java supports multithreading, i.e. the parallel execution of independent program sections. For this purpose, the language itself offers the keywords synchronized
and volatile
- constructs that support the "Monitor & Condition Variable Paradigm" by C. A. R. Hoare. The class library includes further support for parallel programming with threads. Modern JVMs map a Java thread to operating system threads and thus benefit from processors with multiple cores.
Dynamic
Java is structured in such a way that it can be adapted to dynamically changing conditions. Since the modules are linked only at runtime, parts of the software (such as libraries) can be redelivered, for example, without having to adapt the remaining program parts. Interfaces can be used as a basis for communication between two modules; however, the actual implementation can be changed dynamically and, for example, also during runtime.
Object Orientation
The basic idea of object-oriented programming is to combine data and associated functions as closely as possible in a so-called object and to encapsulate them externally (abstraction). The intention behind this is to make large software projects easier to manage and to increase the quality of the software. Another goal of object orientation is a high degree of reusability of software modules.
A new aspect of Java compared to the object-oriented programming languages C++ and Smalltalk is the explicit distinction between interfaces and classes, which is expressed by corresponding keywords interface
and class.
Java does not support inheritance of multiple independent base classes (so-called "multiple inheritance" as in C++ or Eiffel), but it does support the implementation of an arbitrary number of interfaces, which also solves many of the corresponding problems. Method signatures and standard implementations of methods are passed on to the derived classes, but not attributes.
Java is not completely object-oriented, since the basic data types (int, boolean, etc.) are not objects (see also under Java syntax). However, as of Java 5, they are converted automatically and transparently for the programmer into the corresponding object types and vice versa by means of autoboxing, if required.
Example
Source code
Console output
Reflection
Java offers a Reflection API as part of the runtime environment. This makes it possible to access classes and methods at runtime whose existence or exact specification was not known at the time of program creation. This technique is often used in connection with the factory method design pattern.
Annotations
With Java 5, Sun has extended the programming language with annotations. Annotations allow the notation of metadata and enable user-defined language extensions to a certain extent. The purpose of annotations is, among other things, the automatic generation of code and other documents important in software development for recurring patterns based on the shortest possible notes in the source code. Until now, only Javadoc comments with special JavaDoc tags were used for this purpose in Java, which were evaluated by doclets such as the XDoclet.
Annotations can also be contained in the compiled class files. Thus, the source code is not required for their use. In particular, the annotations are also accessible via the Reflection API. For example, they can be used to extend the bean concept.
Modular execution on remote computers
Java provides the ability to write classes that run in different execution environments. For example, applets can be executed in web browsers that support Java. Java's security concept can be used to ensure that unknown classes cannot cause any damage, which is particularly important for applets (see also Sandbox). Examples of Java modules that can be executed in corresponding execution environments are applets, servlets, portlets, MIDlets, xlets, translets, and Enterprise JavaBeans.
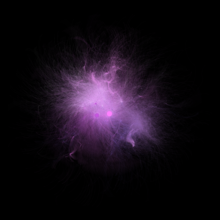

Dependency graph of Java Core classes (created with jdeps and Gephi). In the middle of the diagram you can see the most commonly used classes Object and String.
Features
Object access in Java is implemented VM-internally via references, which are similar to the pointers known from C or C++. The language definition (Java Language Specification) calls them "Reference Values" to make it clear that they are passed in the source code of the respective program as a call by value. For security reasons, these do not allow the actual memory address to be recognized or modified. So-called pointer arithmetic is thus excluded in Java. By design, a common type of error that occurs in other programming languages can thus be excluded from the outset.
Classes that belong together are grouped together in packages. These packages allow limiting the visibility of classes, structuring larger projects, and separating the namespace for different developers. Package names are hierarchical and usually start with the (reverse) Internet domain name of the developer, for example com.google for class libraries provided by Google. Class names only need to be unique within a package. This makes it possible to combine classes from different developers without naming conflicts. However, the hierarchy of package names has no semantic meaning. When it comes to visibility between the classes of two packages, it does not matter where the packages are in the naming hierarchy. Classes are either only visible to classes in their own package, or to all packages.
Furthermore, the language supports threads (concurrently running program parts) and exceptions. Java also includes an automatic garbage collector that removes objects that are no longer referenced from memory.
Java explicitly distinguishes between interfaces and classes. A class can implement any number of interfaces, but always has exactly one base class. Java does not support direct inheritance from multiple classes ("multiple inheritance"), but it does support inheritance over multiple hierarchy levels (class child inherits from class father, which in turn inherits from class grandfather, etc.). Depending on the visibility (public
, protected
, default/package-private, private
), the class inherits methods and attributes (also called fields) from its class ancestors. All classes are derived - directly or indirectly - from the root class Object
.
Java includes an extensive class library. This provides the programmer with a uniform interface (application programming interface, API) that is independent of the underlying operating system.
Java 2 introduced the Java Foundation Classes (JFC), which among other things provide Swing, which is used to create platform-independent graphical user interfaces (GUI) and is based on the Abstract Window Toolkit.
_waving.svg.png)

Duke, the Java Mascot
Questions and Answers
Q: What is the name of the programming language created by Sun Microsystems?
A: The programming language created by Sun Microsystems is called Java.
Q: Who currently supports and keeps Java up to date?
A: Oracle Corporation currently supports and keeps Java up to date.
Q: What are the current long-term support (LTS) versions of Java?
A: The current long-term support (LTS) versions of Java are version 17, 11, and 8.
Q: When was the latest version of Java released?
A: The latest version of Java, version 19, was released in September 2022.
Q: Is there any other company that supports Java besides Oracle?
A: Yes, Eclipse Adoptium also supports Java for at least May 2026 for version 8 and at least September 2027 for version 17.
Q: What type of code does Java use?
A:Java uses object oriented code which means it is based on objects that work together to make programs do their jobs. It looks similar to C, C++ or C# but code written in those languages will not work in most cases without being changed.
Q: How does Java make itself platform independent?
A:Java makes itself platform independent by making the compiler turn code into a special format called bytecode instead of machine code. This means when the program is executed, the bytecode can be interpreted by a special program called a virtual machine which translates it into machine code so it can run on different operating systems such as Android.